Properti nodeName, nodeValue, dan nodeType berisi informasi tentang node.
Section Artikel
Properti Node
Di XML DOM, setiap node adalah objek.
Objek memiliki metode dan properti, yang dapat diakses dan dimanipulasi oleh JavaScript.
Tiga properti node penting adalah:
- nodeName
- nodeValue
- nodeType
Properti nodeName
Properti nodeName menentukan nama sebuah node.
- nodeName bersifat hanya baca
- nodeName dari sebuah node elemen sama dengan nama tag
- nodeName dari node atribut adalah nama atribut
- nodeName dari node teks selalu #teks
- nodeName dari node dokumen selalu #document
Contoh :
<!DOCTYPE html> <html> <body> <p id="demo"></p> <script> var xhttp = new XMLHttpRequest(); xhttp.onreadystatechange = function() { if (this.readyState == 4 && this.status == 200) { myFunction(this); } }; xhttp.open("GET", "books.xml", true); xhttp.send(); function myFunction(xml) { var xmlDoc = xml.responseXML; document.getElementById("demo").innerHTML = xmlDoc.documentElement.nodeName; } </script> </body> </html>
Output :

Properti nodeValue
Properti nodeValue menentukan nilai dari sebuah node.
- nodeValue untuk node elemen tidak ditentukan
- nodeValue untuk node teks adalah teks itu sendiri
- nodeValue untuk node atribut adalah nilai atribut
Dapatkan Nilai dari sebuah Elemen
Kode berikut mengambil nilai simpul teks dari elemen <title> pertama:
Contoh :
<!DOCTYPE html> <html> <body> <p id="demo"></p> <script> var xhttp = new XMLHttpRequest(); xhttp.onreadystatechange = function() { if (this.readyState == 4 && this.status == 200) { myFunction(this); } }; xhttp.open("GET", "books.xml", true); xhttp.send(); function myFunction(xml) { var xmlDoc = xml.responseXML; var x = xmlDoc.getElementsByTagName("title")[0].childNodes[0]; document.getElementById("demo").innerHTML = x.nodeValue; } </script> </body> </html>
Hasil: txt = “Everyday Italian”
Contoh menjelaskan:
- Misalkan kita telah memuat “books.xml” ke xmlDoc
- Dapatkan simpul teks dari simpul elemen <title> pertama
- Tetapkan variabel txt menjadi nilai node teks
Ubah Nilai Elemen
Kode berikut mengubah nilai simpul teks dari elemen <title> pertama:
Contoh :
<!DOCTYPE html> <html> <body> <p id="demo1"></p> <p id="demo2"></p> <script> var xhttp = new XMLHttpRequest(); xhttp.onreadystatechange = function() { if (this.readyState == 4 && this.status == 200) { myFunction(this); } }; xhttp.open("GET", "books.xml", true); xhttp.send(); function myFunction(xml) { var xmlDoc = xml.responseXML; var x; x = xmlDoc.getElementsByTagName("title")[0].childNodes[0]; document.getElementById("demo1").innerHTML = x.nodeValue; x.nodeValue = "Easy Cooking"; x = xmlDoc.getElementsByTagName("title")[0].childNodes[0]; document.getElementById("demo2").innerHTML = x.nodeValue; } </script> </body> </html>
Output :
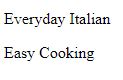
Penjelasan kode
- Misalkan Anda telah memuat “books.xml” ke xmlDoc
- Dapatkan simpul teks dari simpul elemen<title> pertama
- Ubah nilai node teks menjadi “Easy Cooking”.
Properti nodeType
Properti nodeType menentukan jenis node.
nodeType hanya bisa dibaca.
Jenis node yang paling penting adalah, sebgai berikut:
Node type | NodeType |
---|---|
Element | 1 |
Attribute | 2 |
Text | 3 |
Comment | 8 |
Document | 9 |