Section Artikel
Buat Node Elemen Baru
Metode createElement () membuat node elemen baru:
Contoh :
<!DOCTYPE html> <html> <body> <p id="demo"></p> <script> var xhttp = new XMLHttpRequest(); xhttp.onreadystatechange = function() { if (this.readyState == 4 && this.status == 200) { myFunction(this); } }; xhttp.open("GET", "books.xml", true); xhttp.send(); function myFunction(xml) { var x, y, i, newElement, txt, xmlDoc; xmlDoc = xml.responseXML; newElement = xmlDoc.createElement("edition"); x = xmlDoc.getElementsByTagName("book")[0] x.appendChild(newElement); // Display all elements xlen = x.childNodes.length; y = x.firstChild; txt = ""; for (i = 0; i < xlen; i++) { if (y.nodeType == 1) { txt += y.nodeName + "<br>"; } y = y.nextSibling; } document.getElementById("demo").innerHTML = txt; } </script> </body> </html>
Output :

Penjelasan Kode :
- Misalkan “books.xml” dimuat ke xmlDoc
- Buat node elemen <edition> baru
- Tambahkan simpul elemen ke elemen <book> pertama
Buat Node Atribut Baru
CreateAttribute () digunakan untuk membuat node atribut baru:
Contoh :
<!DOCTYPE html> <html> <body> <p id="demo"></p> <script> var xhttp = new XMLHttpRequest(); xhttp.onreadystatechange = function() { if (this.readyState == 4 && this.status == 200) { myFunction(this); } }; xhttp.open("GET", "books.xml", true); xhttp.send(); function myFunction(xml) { var x, newatt, xmlDoc; xmlDoc = xml.responseXML; newatt = xmlDoc.createAttribute("edition"); newatt.nodeValue = "first"; x = xmlDoc.getElementsByTagName("title"); x[0].setAttributeNode(newatt); document.getElementById("demo").innerHTML = "Edition: " + x[0].getAttribute("edition"); } </script> </body> </html>
Output :

Penjelasan Kode :
- Misalkan “books.xml” dimuat ke xmlDoc
- Buat node atribut baru “edition”
- Tetapkan nilai node atribut ke “first”
- Tambahkan node atribut baru ke elemen <title> pertama
Jika atribut sudah ada, itu diganti dengan yang baru.
Buat Atribut Menggunakan setAttribute ()
Karena metode setAttribute () membuat atribut baru jika atribut tidak ada, metode ini dapat digunakan untuk membuat atribut baru.
Contoh :
<!DOCTYPE html> <html> <body> <p id="demo"></p> <script> var xhttp = new XMLHttpRequest(); xhttp.onreadystatechange = function() { if (this.readyState == 4 && this.status == 200) { myFunction(this); } }; xhttp.open("GET", "books.xml", true); xhttp.send(); function myFunction(xml) { var xmlDoc = xml.responseXML; var x = xmlDoc.getElementsByTagName("title"); x[0].setAttribute("edition", "first"); document.getElementById("demo").innerHTML = "Edition: " + x[0].getAttribute("edition"); } </script> </body> </html>
Output :

Penjelasan Kode :
- Misalkan “books.xml” dimuat ke xmlDoc
- Setel nilai atribut “edisi” ke “pertama” untuk elemen <book> pertama
Buat Node Teks
Metode createTextNode () membuat text node baru:
Contoh:
<!DOCTYPE html> <html> <body> <p id="demo"></p> <script> var xhttp = new XMLHttpRequest(); xhttp.onreadystatechange = function() { if (this.readyState == 4 && this.status == 200) { myFunction(this); } }; xhttp.open("GET", "books.xml", true); xhttp.send(); function myFunction(xml) { var xmlDoc = xml.responseXML; var x, y, i, newEle, newText, txt; // add an edition element newEle = xmlDoc.createElement("edition"); newText = xmlDoc.createTextNode("first"); newEle.appendChild(newText); x = xmlDoc.getElementsByTagName("book")[0]; x.appendChild(newEle); // display all elements xlen = x.childNodes.length; y = x.firstChild; txt = ""; for (i = 0; i < xlen; i++) { if (y.nodeType == 1) { txt += y.nodeName + "<br>"; } y = y.nextSibling; } document.getElementById("demo").innerHTML = txt; } </script> </body> </html>
Output:

Penjelasan Kode :
- Misalkan “books.xml” dimuat ke xmlDoc
- Buat node elemen <edition> baru
- Buat node teks baru dengan teks “first”
- Tambahkan node teks baru ke simpul elemen
- Tambahkan node elemen baru ke elemen <book> pertama
Buat Node Bagian CDATA
Metode createCDATASection () membuat node bagian CDATA baru.
Contoh :
<!DOCTYPE html> <html> <body> <p id="demo"></p> <script> var xhttp = new XMLHttpRequest(); xhttp.onreadystatechange = function() { if (this.readyState == 4 && this.status == 200) { myFunction(this); } }; xhttp.open("GET", "books.xml", true); xhttp.send(); function myFunction(xml) { var x, newCDATA, xmlDoc; xmlDoc = xml.responseXML; newCDATA = xmlDoc.createCDATASection("Special Offer & Book Sale"); x = xmlDoc.getElementsByTagName("book")[0]; x.appendChild(newCDATA); document.getElementById("demo").innerHTML = x.lastChild.nodeValue; } </script> </body> </html>
Output:

Penjelasan Kode :
- Misalkan “books.xml” dimuat ke xmlDoc
- Buat node bagian CDATA baru
- Tambahkan simpul CDATA baru ke elemen <book> pertama
Buat Node Komentar
Metode createComment () membuat node komentar baru.
Contoh :
<!DOCTYPE html> <html> <body> <p id="demo"></p> <script> var xhttp = new XMLHttpRequest(); xhttp.onreadystatechange = function() { if (this.readyState == 4 && this.status == 200) { myFunction(this); } }; xhttp.open("GET", "books.xml", true); xhttp.send(); function myFunction(xml) { var x, newComment, xmlDoc; xmlDoc = xml.responseXML; newComment = xmlDoc.createComment("Revised April 2015"); x = xmlDoc.getElementsByTagName("book")[0]; x.appendChild(newComment); document.getElementById("demo").innerHTML = x.lastChild.nodeValue; } </script> </body> </html>
Output :
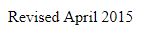
Penjelasan Kode :
- Misalkan “books.xml” dimuat ke xmlDoc
- Buat node komentar baru
- Tambahkan node komentar baru ke elemen <book>pertama