Section Artikel
Tambahkan Node – appendChild ()
Metode appendChild() menambahkan child node ke node yang sudah ada.
Node baru ditambahkan (ditambahkan) setelah node turunan yang ada.
Catatan: Gunakan insertBefore () jika posisi node penting.
Fragmen kode ini membuat elemen (<edition>), dan menambahkannya setelah anak terakhir dari elemen <book> pertama:
Contoh :
<!DOCTYPE html> <html> <body> <p id="demo"></p> <script> var xhttp = new XMLHttpRequest(); xhttp.onreadystatechange = function() { if (this.readyState == 4 && this.status == 200) { myFunction(this); } }; xhttp.open("GET", "books.xml", true); xhttp.send(); function myFunction(xml) { var x, y, i, newElement, txt, xmlDoc; xmlDoc = xml.responseXML; newElement = xmlDoc.createElement("edition"); x = xmlDoc.getElementsByTagName("book")[0] x.appendChild(newElement); // Display all elements xlen = x.childNodes.length; y = x.firstChild; txt = ""; for (i = 0; i < xlen; i++) { if (y.nodeType == 1) { txt += y.nodeName + "<br>"; } y = y.nextSibling; } document.getElementById("demo").innerHTML = txt; } </script> </body> </html>
Output :
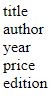
Penjelasan Kode :
- Misalkan “books.xml” dimuat ke xmlDoc
- Buat node baru
- Tambahkan simpul ke elemen <book> pertama
Fragmen kode ini melakukan hal yang sama seperti di atas, tetapi elemen baru ditambahkan dengan nilai:
Contoh :
<!DOCTYPE html> <html> <body> <p id="demo"></p> <script> var xhttp = new XMLHttpRequest(); xhttp.onreadystatechange = function() { if (this.readyState == 4 && this.status == 200) { myFunction(this); } }; xhttp.open("GET", "books.xml", true); xhttp.send(); function myFunction(xml) { var x, y, z, i, xLen, yLen, newEle, newText, xmlDoc, txt; xmlDoc = xml.responseXML; txt = ""; x = xmlDoc.getElementsByTagName("book"); xLen = x.length; for (i = 0; i < xLen; i++) { newEle = xmlDoc.createElement("edition"); newText = xmlDoc.createTextNode("first"); newEle.appendChild(newText); x[i].appendChild(newEle); } // Output all titles and editions y = xmlDoc.getElementsByTagName("title"); yLen = y.length z = xmlDoc.getElementsByTagName("edition"); for (i = 0; i < yLen; i++) { txt += y[i].childNodes[0].nodeValue + " - Edition: " + z[i].childNodes[0].nodeValue + "<br>"; } document.getElementById("demo").innerHTML = txt; } </script> </body> </html>
Output :
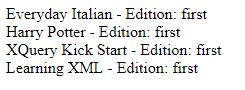
Penjelasan Kode :
- Misalkan “books.xml” dimuat ke xmlDoc
- Buat node baru <edition>
- Buat node teks baru “first”
- Tambahkan text node ke <edition> node
- Tambahkan node <addition> ke elemen <book>
Masukkan Node – insertBefore ()
Metode insertBefore () menyisipkan node sebelum child node yang ditentukan.
Metode ini berguna ketika posisi node yang ditambahkan penting:
Contoh :
<!DOCTYPE html> <html> <body> <p id="demo"></p> <script> var xhttp = new XMLHttpRequest(); xhttp.onreadystatechange = function() { if (this.readyState == 4 && this.status == 200) { myFunction(this); } }; xhttp.open("GET", "books.xml", true); xhttp.send(); function myFunction(xml) { var xmlDoc = xml.responseXML; var newNode = xmlDoc.createElement("book"); var x = xmlDoc.documentElement; var y = xmlDoc.getElementsByTagName("book"); document.getElementById("demo").innerHTML = "Book elements before: " + y.length + "<br>"; x.insertBefore(newNode, y[3]); document.getElementById("demo").innerHTML += "Book elements after: " + y.length; } </script> </body> </html>
Output :
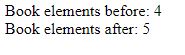
Penjelasan Kode :
- Misalkan “books.xml” dimuat ke xmlDoc
- Buat node elemen <book> baru
- Sisipkan simpul baru di depan node elemen <book> terakhir
Jika parameter kedua dari insertBefore() adalah null, node baru akan ditambahkan setelah last child node yang ada.
x.insertBefore (newNode, null)
dan x.appendChild (newNode)
keduanya akan menambahkan child node baru ke x.
Tambahkan Atribut Baru
Metode setAttribute () menetapkan nilai atribut.
Contoh :
<!DOCTYPE html> <html> <body> <p id="demo"></p> <script> var xhttp = new XMLHttpRequest(); xhttp.onreadystatechange = function() { if (this.readyState == 4 && this.status == 200) { myFunction(this); } }; xhttp.open("GET", "books.xml", true); xhttp.send(); function myFunction(xml) { var xmlDoc = xml.responseXML; var x = xmlDoc.getElementsByTagName("title"); x[0].setAttribute("edition", "first"); document.getElementById("demo").innerHTML = "Edition: " + x[0].getAttribute("edition"); } </script> </body> </html>
Output :
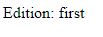
Penjelasan Kode :
- Misalkan “books.xml” telah dimuat ke xmlDoc
- Setel nilai atribut “edition” ke “first” untuk elemen <book> pertama
Tidak ada metode yang disebut add Attribute ()
SetAttribute() akan membuat atribut baru jika atribut tidak ada.
Catatan: Jika atribut sudah ada, metode setAttribute () akan menimpa nilai yang sudah ada.
Menambahkan Teks ke Node Teks – insertData()
Metode insertData() memasukkan data ke dalam node teks yang ada.
Metode insertData() memiliki dua parameter:
- offset – Di mana mulai memasukkan karakter (dimulai dari nol)
- string – String yang akan disisipkan
Fragmen kode berikut akan menambahkan “Easy” ke text node dari elemen <title> pertama dari XML yang dimuat:
Contoh :
<!DOCTYPE html> <html> <body> <p id="demo"></p> <script> var xhttp = new XMLHttpRequest(); xhttp.onreadystatechange = function() { if (this.readyState == 4 && this.status == 200) { myFunction(this); } }; xhttp.open("GET", "books.xml", true); xhttp.send(); function myFunction(xml) { var x, txt, xmlDoc; xmlDoc = xml.responseXML; x = xmlDoc.getElementsByTagName("title")[0].childNodes[0]; txt = x.nodeValue + "<br>"; x.insertData(0,"Easy "); txt += x.nodeValue; document.getElementById("demo").innerHTML = txt; } </script> </body> </html>
Output :
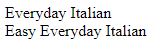