Metode removeChild () menghapus node tertentu.
Metode removeAttribute () menghapus atribut tertentu.
Section Artikel
Hapus sebuah Element Node
Metode removeChild() menghapus node tertentu.
Saat sebuah node dihapus, semua node turunannya juga dihapus.
Kode ini akan menghapus elemen <book> pertama dari xml yang dimuat:
Contoh :
<!DOCTYPE html> <html> <body> <p id="demo"></p> <script> var xhttp = new XMLHttpRequest(); xhttp.onreadystatechange = function() { if (this.readyState == 4 && this.status == 200) { myFunction(this); } }; xhttp.open("GET", "books.xml", true); xhttp.send(); function myFunction(xml) { var xmlDoc = xml.responseXML; var root = xmlDoc.documentElement; var currNode = root.childNodes[1]; removedNode = currNode.removeChild(currNode.childNodes[1]); document.getElementById("demo").innerHTML = "Removed node: " + removedNode.nodeName; } </script> </body> </html>
Output :

Penjelasan Kode :
- Misalkan “books.xml” dimuat ke xmlDoc
- Atur variabel y menjadi simpul elemen yang akan dihapus
- Hapus node elemen dengan menggunakan metode removeChild() dari parents node.
Hapus Sendiri – Remove Current Node
Metode removeChild() adalah satu-satunya cara untuk menghapus node tertentu.
Ketika kita telah menavigasi ke node yang ingin kita hapus, maka untuk menghapus node tersebut menggunakan properti parentNode dan metode removeChild ():
Contoh :
<!DOCTYPE html> <html> <body> <p id="demo"></p> <script> var xhttp = new XMLHttpRequest(); xhttp.onreadystatechange = function() { if (this.readyState == 4 && this.status == 200) { myFunction(this); } }; xhttp.open("GET", "books.xml", true); xhttp.send(); function myFunction(xml) { var x, xmlDoc, txt; xmlDoc = xml.responseXML; txt = "Number of book nodes before removeChild(): " + xmlDoc.getElementsByTagName("book").length + "<br>"; x = xmlDoc.getElementsByTagName("book")[0]; x.parentNode.removeChild(x); txt += "Number of book nodes after removeChild(): " + xmlDoc.getElementsByTagName("book").length; document.getElementById("demo").innerHTML = txt; } </script> </body> </html>
Output :

Penjelasan Kode :
- Misalkan “books.xml” dimuat ke xmlDoc
- Atur variabel y menjadi simpul elemen yang akan dihapus
- Hapus node elemen dengan menggunakan properti parentNode dan metode removeChild()
Remove Node Teks
Metode removeChild() juga dapat digunakan untuk menghapus node teks:
Contoh :
<!DOCTYPE html> <html> <body> <p id="demo"></p> <script> var xhttp = new XMLHttpRequest(); xhttp.onreadystatechange = function() { if (this.readyState == 4 && this.status == 200) { myFunction(this); } }; xhttp.open("GET", "books.xml", true); xhttp.send(); function myFunction(xml) { var x, y, xmlDoc, txt; xmlDoc = xml.responseXML; txt = ""; x = xmlDoc.getElementsByTagName("title")[0]; txt += "Child nodes: " + x.childNodes.length +"<br>"; y = x.childNodes[0]; x.removeChild(y); txt += "Child nodes: " + x.childNodes.length; document.getElementById("demo").innerHTML = txt; } </script> </body> </html>
Output ;

Penjelasan Kode :
- Misalkan “books.xml” dimuat ke xmlDoc
- Tetapkan variabel x menjadi simpul elemen judul pertama
- Atur variabel y menjadi simpul teks yang akan dihapus
- Hapus node elemen dengan menggunakan metode removeChild () dari parents node
Tidak umum menggunakan removeChild() hanya untuk menghapus teks dari node. Properti nodeValue dapat digunakan sebagai gantinya.
Clear Node Teks
Properti nodeValue dapat digunakan untuk mengubah nilai node teks:
Contoh :
<!DOCTYPE html> <html> <body> <p id="demo"></p> <script> var xhttp = new XMLHttpRequest(); xhttp.onreadystatechange = function() { if (this.readyState == 4 && this.status == 200) { myFunction(this); } }; xhttp.open("GET", "books.xml", true); xhttp.send(); function myFunction(xml) { var x, y, xmlDoc, txt; xmlDoc = xml.responseXML; txt = ""; x = xmlDoc.getElementsByTagName("title")[0]; txt += "Child nodes: " + x.childNodes.length +"<br>"; y = x.childNodes[0]; x.removeChild(y); txt += "Child nodes: " + x.childNodes.length; document.getElementById("demo").innerHTML = txt; } </script> </body> </html>
Output :

Penjelasan Kode :
- Misalkan “books.xml” dimuat ke xmlDoc
- Dapatkan first node child dari elemen judul pertama.
- Gunakan properti nodeValue untuk menghapus teks dari text node.
Hapus sebuah Node Atribut berdasarkan Nama
Metode removeAttribute() menghapus node atribut dengan namanya.
Contoh: removeAttribute (‘category’)
Kode ini menghapus atribut “kategori” di elemen <book> pertama:
Contoh :
<!DOCTYPE html> <html> <body> <p id="demo"></p> <script> var xhttp = new XMLHttpRequest(); xhttp.onreadystatechange = function() { if (this.readyState == 4 && this.status == 200) { myFunction(this); } }; xhttp.open("GET", "books.xml", true); xhttp.send(); function myFunction(xml) { var xmlDoc = xml.responseXML; var x = xmlDoc.getElementsByTagName("book"); document.getElementById("demo").innerHTML = x[0].getAttribute('category') + "<br>"; x[0].removeAttribute('category'); document.getElementById("demo").innerHTML += x[0].getAttribute('category'); } </script> </body> </html>
Output :

Penjelasan Kode :
- Misalkan “books.xml” dimuat ke xmlDoc
- Gunakan getElementsByTagName () untuk mendapatkan node buku
- Hapus atribut “category” dari node elemen buku pertama
Hapus Atribut Node berdasarkan Objek
Metode removeAttributeNode () menghapus node atribut, menggunakan objek node sebagai parameter.
Contoh: removeAttributeNode (x)
Kode ini menghapus semua atribut dari semua elemen <book> :
Contoh :
<!DOCTYPE html> <html> <body> <p id="demo"></p> <script> var xhttp = new XMLHttpRequest(); xhttp.onreadystatechange = function() { if (this.readyState == 4 && this.status == 200) { myFunction(this); } }; xhttp.open("GET", "books.xml", true); xhttp.send(); function myFunction(xml) { var x, i, attnode, old_att, xmlDoc, txt; xmlDoc = xml.responseXML; txt = ""; x = xmlDoc.getElementsByTagName('book'); for (i = 0; i < x.length; i++) { while (x[i].attributes.length > 0) { attnode = x[i].attributes[0]; old_att = x[i].removeAttributeNode(attnode); txt += "Removed: " + old_att.nodeName + ": " + old_att.nodeValue + "<br>"; } } document.getElementById("demo").innerHTML = txt; } </script> </body> </html>
Output :
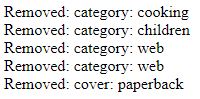
Penjelasan Kode :
- Misalkan “books.xml” dimuat ke xmlDoc
- Gunakan getElementsByTagName () untuk mendapatkan semua node buku
- Untuk setiap elemen buku, periksa apakah ada atribut
- Meskipun ada atribut dalam elemen buku, hapus atribut tersebut