Metode replaceChild() menggantikan node yang ditentukan.
Properti nodeValue menggantikan teks dalam node teks.
Section Artikel
Replace Node Elemen
Metode replaceChild () digunakan untuk mengganti node.
Fragmen kode berikut menggantikan elemen pertama:
Contoh :
<!DOCTYPE html> <html> <body> <p id="demo"></p> <script> var xhttp = new XMLHttpRequest(); xhttp.onreadystatechange = function() { if (this.readyState == 4 && this.status == 200) { myFunction(this); } }; xhttp.open("GET", "books.xml", true); xhttp.send(); function myFunction(xml) { var x, y, z, i, newNode, newTitle, newText, xmlDoc, txt; xmlDoc = xml.responseXML; txt = ""; x = xmlDoc.documentElement; // Create a book element, title element and a text node newNode = xmlDoc.createElement("book"); newTitle = xmlDoc.createElement("title"); newText = xmlDoc.createTextNode("A Notebook"); // Add a text node to the title node newTitle.appendChild(newText); // Add the title node to the book node newNode.appendChild(newTitle); y = xmlDoc.getElementsByTagName("book")[0]; // Replace the first book node with the new book node x.replaceChild(newNode, y); z = xmlDoc.getElementsByTagName("title"); // Output all titles for (i = 0; i < z.length; i++) { txt += z[i].childNodes[0].nodeValue + "<br>"; } document.getElementById("demo").innerHTML = txt; } </script> </body> </html>
Output :
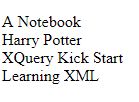
Penjelasan Kode :
- Muat “books.xml” ke xmlDoc
- Buat node elemen <book> baru
- Buat node elemen <title> baru
- Buat node teks baru dengan teks “A Notebook”
- Tambahkan node teks baru ke node elemen baru <title>
- Tambahkan node elemen baru <title> ke node elemen baru <book>
- Ganti simpul elemen <book> pertama dengan simpul elemen <book> baru
Ganti Data di Node Teks
Metode replaceData() digunakan untuk mengganti data dalam node teks.
Metode replaceData() memiliki tiga parameter:
- offset – Tempat mulai mengganti karakter. Nilai offset dimulai dari nol
- length – Banyak karakter yang akan diganti
- string – String yang akan disisipkan
Contoh :
<!DOCTYPE html> <html> <body> <p id="demo"></p> <script> var xhttp = new XMLHttpRequest(); xhttp.onreadystatechange = function() { if (this.readyState == 4 && this.status == 200) { myFunction(this); } }; xhttp.open("GET", "books.xml", true); xhttp.send(); function myFunction(xml) { var xmlDoc = xml.responseXML; var x = xmlDoc.getElementsByTagName("title")[0].childNodes[0]; document.getElementById("demo").innerHTML = x.nodeValue; x.replaceData(0, 8, "Easy"); document.getElementById("demo").innerHTML += "<br>" + x.nodeValue; } </script> </body> </html>
Output :
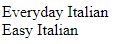
Penjelasan Kode :
- Muat “books.xml” ke xmlDoc
- Dapatkan simpul teks dari simpul elemen <title> pertama
- Gunakan metode replaceData untuk mengganti delapan karakter pertama dari node teks dengan “Easy”
Gunakan Properti nodeValue Sebagai ganti
Lebih mudah untuk mengganti data dalam node teks menggunakan properti nodeValue.
Fragmen kode berikut akan menggantikan nilai text node di elemen <title> pertama dengan “Easy Italian”:
Contoh :
<!DOCTYPE html> <html> <body> <p id="demo"></p> <script> var xhttp = new XMLHttpRequest(); xhttp.onreadystatechange = function() { if (this.readyState == 4 && this.status == 200) { myFunction(this); } }; xhttp.open("GET", "books.xml", true); xhttp.send(); function myFunction(xml) { var x, xmlDoc, txt; xmlDoc = xml.responseXML; x = xmlDoc.getElementsByTagName("title")[0].childNodes[0]; txt = x.nodeValue + "<br>"; x.nodeValue="Easy Italian"; txt += x.nodeValue; document.getElementById("demo").innerHTML = txt } </script> </body> </html>
Output :
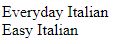
Penjelasan Kode :
- Muat “books.xml” ke xmlDoc
- Dapatkan text node dari simpul elemen <title> pertama
- Gunakan properti nodeValue untuk mengubah teks dari node teks