Properti nodeValue digunakan untuk mendapatkan nilai teks dari sebuah node.
Metode getAttribute() mengembalikan nilai atribut.
Section Artikel
Dapatkan Nilai dari sebuah Elemen
Di DOM, semuanya adalah node. Node elemen tidak memiliki nilai teks.
Nilai teks dari node elemen disimpan di child node. Node ini disebut node teks.
Untuk mengambil nilai teks suatu elemen, kita harus mengambil nilai dari node teks elemen tersebut.
Metode getElementsByTagName
Metode getElementsByTagName()
mengembalikan daftar node dari semua elemen dengan nama tag yang ditentukan dalam urutan yang sama seperti yang muncul di dokumen sumber.
Misalkan “books.xml” telah dimuat ke xmlDoc.
Kode ini mengambil elemen <title> pertama:
var x = xmlDoc.getElementsByTagName("title")[0];
Properti ChildNodes
Properti childNodes mengembalikan daftar child node elemen.
Kode berikut mengambil simpul teks dari elemen <title> pertama:
x = xmlDoc.getElementsByTagName("title")[0]; y = x.childNodes[0];
Properti nodeValue
Properti nodeValue mengembalikan texs value dari texs node.
Kode berikut mengambil nilai teks dari text node dari elemen<title> pertama:
Contoh :
x = xmlDoc.getElementsByTagName("title")[0]; y = x.childNodes[0]; z = y.nodeValue;
Output z: “Everyday Italian”
Contoh Keseluruh dari contoh Node Value
<!DOCTYPE html> <html> <body> <p id="demo"></p> <script> var xhttp = new XMLHttpRequest(); xhttp.onreadystatechange = function() { if (this.readyState == 4 && this.status == 200) { myFunction(this); } }; xhttp.open("GET", "books.xml", true); xhttp.send(); function myFunction(xml) { var xmlDoc = xml.responseXML; var x = xmlDoc.getElementsByTagName('title')[0]; var y = x.childNodes[0]; document.getElementById("demo").innerHTML = y.nodeValue; } </script> </body> </html>
Output :
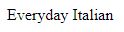
Dapatkan Nilai dari suatu Atribut
Di DOM, atribut adalah node. Tidak seperti node elemen, node atribut memiliki text value.
Cara mendapatkan nilai suatu atribut adalah dengan mendapatkan text value nya.
Hal ini bisa dilakukan dengan menggunakan metode getAttribute() atau menggunakan properti nodeValue dari node atribut.
Dapatkan Nilai Atribut – getAttribute ()
Metode getAttribute() mengembalikan nilai atribut.
Kode berikut mengambil nilai teks dari atribut “lang” dari elemen <title> pertama:
Contoh :
<!DOCTYPE html> <html> <body> <p id="demo"></p> <script> var xhttp = new XMLHttpRequest(); xhttp.onreadystatechange = function() { if (this.readyState == 4 && this.status == 200) { myFunction(this); } }; xhttp.open("GET", "books.xml", true); xhttp.send(); function myFunction(xml) { var x, i, xmlDoc, txt; xmlDoc = xml.responseXML; txt = ""; x = xmlDoc.getElementsByTagName('book'); for (i = 0; i < x.length; i++) { txt += x[i].getAttribute('category') + "<br>"; } document.getElementById("demo").innerHTML = txt; }</script> </body> </html>
Output :

Dapatkan Nilai Atribut – getAttributeNode ()
Metode getAttributeNode() mengembalikan node atribut.
Kode berikut mengambil txt value dari atribut “lang” dari elemen <title> pertama:
Contoh :
<!DOCTYPE html> <html> <body> <p id="demo"></p> <script> var xhttp = new XMLHttpRequest(); xhttp.onreadystatechange = function() { if (this.readyState == 4 && this.status == 200) { myFunction(this); } }; xhttp.open("GET", "books.xml", true); xhttp.send(); function myFunction(xml) { var xmlDoc = xml.responseXML; var x = xmlDoc.getElementsByTagName("title")[0]; var y = x.getAttributeNode("lang"); var txt = y.nodeValue; document.getElementById("demo").innerHTML = txt; } </script> </body> </html>
Output :
