Properti nodeValue digunakan untuk mengubah node value.
Metode setAttribute () digunakan untuk mengubah nilai atribut.
Section Artikel
Ubah Nilai Elemen
Di DOM, semuanya adalah node. Node elemen tidak memiliki text value.
Text value dari node elemen disimpan di child node. Node ini disebut text node.
Untuk mengubah nilai teks suatu elemen, kita harus mengubah value dari node teks elemen tersebut.
Ubah Nilai Node Teks
Properti nodeValue dapat digunakan untuk mengubah nilai node teks.
Misalkan “books.xml” telah dimuat ke xmlDoc.
Kode ini mengubah nilai simpul teks dari elemen <title> pertama:
Contoh :
<!DOCTYPE html> <html> <body> <p id="demo"></p> <script> var xhttp = new XMLHttpRequest(); xhttp.onreadystatechange = function() { if (this.readyState == 4 && this.status == 200) { myFunction(this); } }; xhttp.open("GET", "books.xml", true); xhttp.send(); function myFunction(xml) { var xmlDoc = xml.responseXML; var x; var txt = ""; x = xmlDoc.getElementsByTagName("title")[0].childNodes[0]; txt += x.nodeValue + "<br>"; x.nodeValue = "Easy Cooking"; x = xmlDoc.getElementsByTagName("title")[0].childNodes[0]; txt += x.nodeValue + "<br>"; document.getElementById("demo").innerHTML = txt; } </script> </body> </html>
Output :
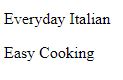
Penjelasan Kode :
- Misalkan “books.xml” dimuat ke xmlDoc
- Dapatkan simpul anak pertama dari elemen <title>
- Ubah nilai node menjadi “new content”
Ubah Nilai Atribut
Di DOM, atribut adalah node. Tidak seperti node elemen, node atribut memiliki text value.
Cara untuk mengubah nilai atribut adalah dengan mengubah text value nya.
Hal ini bisa dilakukan dengan menggunakan metode setAttribute() atau menyetel properti nodeValue dari node atribut.
Ubah Atribut Menggunakan setAttribute ()
Metode setAttribute () mengubah nilai atribut.
Jika atribut tidak ada, atribut baru dibuat.
Kode ini mengubah atribut kategori dari elemen <book> :
Contoh :
<!DOCTYPE html> <html> <body> <p id="demo"></p> <script> var xhttp = new XMLHttpRequest(); xhttp.onreadystatechange = function() { if (this.readyState == 4 && this.status == 200) { myFunction(this); } }; xhttp.open("GET", "books.xml", true); xhttp.send(); function myFunction(xml) { var xmlDoc = xml.responseXML; var x = xmlDoc.getElementsByTagName('book'); x[0].setAttribute("category","food"); document.getElementById("demo").innerHTML = x[0].getAttribute("category"); } </script> </body> </html>
Penjelasan Kode :
- Misalkan “books.xml” dimuat ke xmlDoc
- Dapatkan elemen <book> pertama
- Ubah nilai atribut “category” menjadi “food”
Catatan: Jika atribut tidak ada, atribut baru dibuat (dengan nama dan nilai ditentukan).
Ubah Atribut Menggunakan nodeValue
Properti nodeValue adalah nilai dari node atribut.
Mengubah properti nilai mengubah nilai atribut.
Contoh :
<!DOCTYPE html> <html> <body> <p id="demo"></p> <script> var xhttp = new XMLHttpRequest(); xhttp.onreadystatechange = function() { if (this.readyState == 4 && this.status == 200) { myFunction(this); } }; xhttp.open("GET", "books.xml", true); xhttp.send(); function myFunction(xml) { var xmlDoc = xml.responseXML; var x = xmlDoc.getElementsByTagName("book")[0] var y = x.getAttributeNode("category"); var txt = y.nodeValue + "<br>"; y.nodeValue ="food"; txt += y.nodeValue; document.getElementById("demo").innerHTML = txt; } </script> </body> </html>
Output :

Penjelasan Kode :
- Misalkan “books.xml” dimuat ke xmlDoc
- Dapatkan atribut “category” dari elemen <book> pertama
- Ubah nilai node atribut menjadi “food”